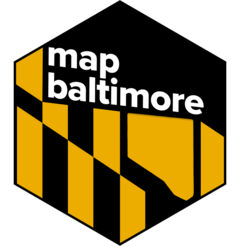
Get area 311 service requests from Open Baltimore
Source:R/get_area_requests.R
get_area_requests.Rd
Get 311 service requests for a specific area. Service requests from 2017 to 2020 area available but only a single year can be requested at a time. Duplicate requests are removed from the returned data. Requests can be filtered by request type, responsible city agency, or both. You can return multiple types or agencies, by using a custom where query parameter or by calling each type/agency separately.
Usage
get_area_requests(
area = NULL,
year = 2022,
date_range = NULL,
request_type = NULL,
agency = NULL,
where = NULL,
dist = NULL,
diag_ratio = NULL,
unit = "m",
asp = NULL,
trim = FALSE,
geometry = TRUE,
crs = pkgconfig::get_config("mapbaltimore.crs", 2804),
duplicates = FALSE,
...
)
Arguments
- area
sf, sfc, or bbox object. If multiple areas are provided, they are unioned into a single sf object using
sf::st_union()
.- year
Year for service requests. Default 2021. 2017 to 2022 supported.
- date_range
Date range as character vector in format of c("YYYY-MM-DD", "YYYY-MM-DD"). Minimum and maximum values are used if length is greater than 1.
- request_type
Service request type.
- agency
City agency responsible for request. Options include "Transportation", "BGE", "Solid Waste", "Housing", "Water Wastewater", "Health", "Call Center", "Finance", "Liquor Board", "Recreation & Parks", "Fire Department", "Parking Authority", and "General Services"
- where
string for where condition. This parameter is ignored if a request_type or agency are provided.
- dist
buffer distance in units. Optional.
- diag_ratio
ratio of diagonal distance of area's bounding box used as buffer distance. e.g. if the diagonal distance is 3000 meters and the "diag_ratio = 0.1" a 300 meter will be used. Ignored when
dist
is provided.- unit
Units for buffer. Supported options include "meter", "foot", "kilometer", and "mile", "nautical mile" Common abbreviations (e.g. "km" instead of "kilometer") are also supported. Distance in units is converted to units matching GDAL units for x; defaults to "meter"
- asp
Aspect ratio of width to height as a numeric value (e.g. 0.33) or character (e.g. "1:3"). If numeric,
get_asp()
returns the same value without modification.- trim
If
TRUE
, x is trimmed to y withst_trim()
.- geometry
Default
TRUE.
IfFALSE
, return requests with missing latitude/longitude (for years prior to 2021 only).- crs
Cordinate reference system to return, Default: 4326 for
sf_to_df()
andNULL
fordf_to_sf()
.- duplicates
If
TRUE
, return 311 service requests marked as "Duplicate". IfFALSE
, filter duplicate requests out of results.- ...
Arguments passed on to
esri2sf::esri2sf
outFields
vector of fields you want to include. default is
NULL
for all fields.replaceDomainInfo
If
TRUE
, add domain information to the return data frame. DefaultFALSE
.
Examples
# Get boundary for Edmondson Village
area <- get_area("neighborhood", "Edmondson Village")
# Get fallen limb requests for 2022
get_area_requests(
area = area,
date_range = c("2022-11-01", "2022-12-31"),
request_type = "FOR-Fallen Limb"
)
#> ── Downloading "Customer_Service_Request311_2021_Present" from <https://egis.bal
#> Layer type: "Feature Layer"
#> Geometry type: "esriGeometryPoint"
#> Service CRS: "EPSG:2248"
#> Output CRS: "EPSG:2804"
#>
#> Simple feature collection with 3 features and 21 fields
#> Geometry type: POINT
#> Dimension: XY
#> Bounding box: xmin: 426988.6 ymin: 181491.5 xmax: 427213.4 ymax: 181615.3
#> Projected CRS: NAD83(HARN) / Maryland
#> service_request_num sr_type method_received created_date
#> 1 22-00893386 FOR-Fallen Limb Phone 2022-11-11 18:16:36
#> 2 22-00926488 FOR-Fallen Limb Phone 2022-11-27 14:20:02
#> 3 22-00990947 FOR-Fallen Limb Phone 2022-12-23 11:36:22
#> sr_status sr_status_url
#> 1 Closed https://balt311.baltimorecity.gov/citizen/requests/22-00893386
#> 2 Closed https://balt311.baltimorecity.gov/citizen/requests/22-00926488
#> 3 Closed https://balt311.baltimorecity.gov/citizen/requests/22-00990947
#> status_date due_date close_date
#> 1 2022-11-14 09:36:55 2022-11-14 18:16:36 2022-11-14 09:36:55
#> 2 2023-01-11 06:04:40 2022-11-28 14:20:02 2023-01-11 06:04:40
#> 3 2023-04-21 18:28:49 2022-12-26 11:36:21 2023-04-21 18:28:49
#> agency last_activity
#> 1 Recreation & Parks Service Response
#> 2 Recreation & Parks Service Response
#> 3 Recreation & Parks Service Response
#> last_activity_date outcome
#> 1 2022-11-14 09:36:55 SR closed by agency-specific work management system
#> 2 2023-01-11 06:04:40 SR closed by agency-specific work management system
#> 3 2023-04-21 18:28:49 SR closed by agency-specific work management system
#> days_to_close address zip_code neighborhood
#> 1 2.64 1303 N WOODINGTON RD 21229 Edmondson Village
#> 2 44.66 1309 N WOODINGTON RD 21229 Edmondson Village
#> 3 119.29 4114 MOUNTWOOD RD 21229 Edmondson Village
#> council_district police_district latitude longitude geometry
#> 1 8 Southwestern 39.30233 -76.68711 POINT (426988.6 181615.3)
#> 2 8 Southwestern 39.30230 -76.68657 POINT (427034.9 181612.2)
#> 3 8 Southwestern 39.30121 -76.68450 POINT (427213.4 181491.5)
# Get dirty alley service requests for multiple years using purrr::map_dfr()
purrr::list_rbind(
purrr::map(
c(2021, 2020),
~ get_area_requests(
area = area,
year = .x,
request_type = "SW-Dirty Alley"
)
)
)
#> ── Downloading "Customer_Service_Request311_2021_Present" from <https://egis.bal
#> Layer type: "Feature Layer"
#> Geometry type: "esriGeometryPoint"
#> Service CRS: "EPSG:2248"
#> Output CRS: "EPSG:2804"
#>
#> ℹ Removing 1 duplicate 311 service request.
#> ── Downloading "311_Customer_Service_Requests_2020.csv" from <https://opendata.b
#> Layer type: "Table"
#>
#> ℹ Removing 5 duplicate 311 service requests.
#> service_request_num sr_type method_received created_date
#> 1 21-00053788 SW-Dirty Alley Phone 2021-01-22 08:07:54
#> 2 21-00059271 SW-Dirty Alley Phone 2021-01-24 16:54:01
#> 3 21-00051584 SW-Dirty Alley API 2021-01-21 13:36:09
#> 4 21-00107202 SW-Dirty Alley Phone 2021-02-12 08:57:40
#> 5 21-00110903 SW-Dirty Alley Phone 2021-02-13 10:44:24
#> 6 21-00115345 SW-Dirty Alley API 2021-02-15 11:46:25
#> 7 21-00142040 SW-Dirty Alley Phone 2021-02-24 14:37:26
#> 8 21-00062237 SW-Dirty Alley Phone 2021-01-25 15:55:46
#> 9 21-00139354 SW-Dirty Alley API 2021-02-23 22:06:52
#> 10 21-00070593 SW-Dirty Alley System 2021-01-28 13:57:46
#> 11 21-00100906 SW-Dirty Alley API 2021-02-10 08:01:58
#> 12 21-00153184 SW-Dirty Alley API 2021-02-28 12:44:37
#> 13 21-00155352 SW-Dirty Alley API 2021-03-01 12:38:49
#> 14 21-00159174 SW-Dirty Alley API 2021-03-02 13:45:11
#> 15 21-00172421 SW-Dirty Alley API 2021-03-07 16:09:48
#> 16 21-00185891 SW-Dirty Alley Phone 2021-03-11 16:08:06
#> 17 21-00204853 SW-Dirty Alley Phone 2021-03-18 17:11:50
#> 18 21-00215385 SW-Dirty Alley Phone 2021-03-23 11:48:32
#> 19 21-00245245 SW-Dirty Alley API 2021-04-03 17:09:57
#> 20 21-00245269 SW-Dirty Alley API 2021-04-03 17:21:35
#> 21 21-00248633 SW-Dirty Alley API 2021-04-05 16:20:02
#> 22 21-00262746 SW-Dirty Alley Phone 2021-04-09 16:27:50
#> 23 21-00254836 SW-Dirty Alley Phone 2021-04-07 13:28:43
#> 24 21-00279074 SW-Dirty Alley System 2021-04-15 16:01:26
#> 25 21-00307201 SW-Dirty Alley System 2021-04-26 13:55:37
#> 26 21-00323235 SW-Dirty Alley Phone 2021-05-01 09:30:44
#> 27 21-00357914 SW-Dirty Alley API 2021-05-13 12:31:49
#> 28 21-00383219 SW-Dirty Alley Phone 2021-05-21 15:15:30
#> 29 21-00383184 SW-Dirty Alley Phone 2021-05-21 15:12:51
#> 30 21-00383142 SW-Dirty Alley Phone 2021-05-21 15:09:15
#> 31 21-00383160 SW-Dirty Alley Phone 2021-05-21 15:10:28
#> 32 21-00383210 SW-Dirty Alley Phone 2021-05-21 15:14:26
#> 33 21-00383863 SW-Dirty Alley Phone 2021-05-21 19:21:21
#> 34 21-00382017 SW-Dirty Alley Phone 2021-05-21 11:12:17
#> 35 21-00396283 SW-Dirty Alley API 2021-05-26 15:05:24
#> 36 21-00406083 SW-Dirty Alley Phone 2021-05-30 12:44:57
#> 37 21-00424359 SW-Dirty Alley API 2021-06-06 13:09:04
#> 38 21-00456861 SW-Dirty Alley Phone 2021-06-17 11:19:24
#> 39 21-00468460 SW-Dirty Alley Phone 2021-06-22 11:44:24
#> 40 21-00460319 SW-Dirty Alley Phone 2021-06-18 13:11:34
#> 41 21-00498057 SW-Dirty Alley Phone 2021-07-02 11:04:07
#> 42 21-00500273 SW-Dirty Alley Phone 2021-07-02 18:48:40
#> 43 21-00518225 SW-Dirty Alley Phone 2021-07-09 15:28:32
#> 44 21-00526294 SW-Dirty Alley Phone 2021-07-13 11:11:08
#> 45 21-00544733 SW-Dirty Alley Phone 2021-07-19 17:37:31
#> 46 21-00602168 SW-Dirty Alley Phone 2021-08-09 14:43:25
#> 47 21-00653598 SW-Dirty Alley Phone 2021-08-26 15:57:45
#> 48 21-00679949 SW-Dirty Alley API 2021-09-06 07:29:58
#> 49 21-00704816 SW-Dirty Alley API 2021-09-15 11:44:24
#> 50 21-00742844 SW-Dirty Alley Phone 2021-09-28 18:49:15
#> 51 21-00759532 SW-Dirty Alley Phone 2021-10-01 13:05:37
#> 52 21-00785583 SW-Dirty Alley Phone 2021-10-11 10:52:18
#> 53 21-00775404 SW-Dirty Alley Phone 2021-10-07 09:35:42
#> 54 21-00718065 SW-Dirty Alley Phone 2021-09-20 10:06:08
#> 55 21-00729023 SW-Dirty Alley Phone 2021-09-23 12:37:19
#> 56 21-00720343 SW-Dirty Alley Phone 2021-09-20 19:26:57
#> 57 21-00731337 SW-Dirty Alley Phone 2021-09-24 09:41:59
#> 58 21-00725990 SW-Dirty Alley Phone 2021-09-22 13:00:02
#> 59 21-00725995 SW-Dirty Alley Phone 2021-09-22 13:01:30
#> 60 21-00726393 SW-Dirty Alley Phone 2021-09-22 14:46:02
#> 61 21-00834537 SW-Dirty Alley API 2021-10-28 13:40:39
#> 62 21-00853412 SW-Dirty Alley Phone 2021-11-04 15:15:30
#> 63 21-00866979 SW-Dirty Alley Phone 2021-11-09 16:07:05
#> 64 21-00888749 SW-Dirty Alley Phone 2021-11-17 14:57:40
#> 65 21-00896245 SW-Dirty Alley Phone 2021-11-20 11:44:50
#> 66 21-00924055 SW-Dirty Alley Phone 2021-12-02 15:38:46
#> 67 21-00928778 SW-Dirty Alley Phone 2021-12-04 13:01:24
#> 68 21-00941216 SW-Dirty Alley Phone 2021-12-08 14:50:21
#> 69 21-00941211 SW-Dirty Alley Phone 2021-12-08 14:49:14
#> 70 21-00944073 SW-Dirty Alley Phone 2021-12-09 13:50:59
#> 71 21-00948827 SW-Dirty Alley Phone 2021-12-11 09:53:47
#> 72 21-00948939 SW-Dirty Alley Phone 2021-12-11 10:54:17
#> 73 21-00969415 SW-Dirty Alley Phone 2021-12-20 08:44:52
#> 74 21-00975849 SW-Dirty Alley Phone 2021-12-22 13:46:20
#> 75 20-00009981 SW-Dirty Alley API 2020-01-06 22:34:50
#> 76 20-00042742 SW-Dirty Alley API 2020-01-23 14:19:56
#> 77 20-00108512 SW-Dirty Alley API 2020-02-23 18:13:18
#> 78 20-00143990 SW-Dirty Alley Phone 2020-03-11 10:04:25
#> 79 20-00150539 SW-Dirty Alley Phone 2020-03-13 14:26:56
#> 80 20-00186619 SW-Dirty Alley Phone 2020-03-24 10:56:30
#> 81 20-00242451 SW-Dirty Alley Phone 2020-04-28 13:29:55
#> 82 20-00256369 SW-Dirty Alley Phone 2020-05-06 10:43:39
#> 83 20-00256372 SW-Dirty Alley Phone 2020-05-06 10:45:18
#> 84 20-00217790 SW-Dirty Alley Phone 2020-04-13 09:58:47
#> 85 20-00292887 SW-Dirty Alley Phone 2020-05-26 13:56:24
#> 86 20-00378707 SW-Dirty Alley Phone 2020-06-23 13:56:29
#> 87 20-00395207 SW-Dirty Alley Phone 2020-06-29 14:55:17
#> 88 20-00411382 SW-Dirty Alley Phone 2020-07-03 15:58:51
#> 89 20-00411388 SW-Dirty Alley Phone 2020-07-03 16:00:39
#> 90 20-00398279 SW-Dirty Alley Phone 2020-06-30 12:24:50
#> 91 20-00401125 SW-Dirty Alley Phone 2020-07-01 08:47:37
#> 92 20-00491791 SW-Dirty Alley Phone 2020-07-30 15:23:40
#> 93 20-00513600 SW-Dirty Alley Phone 2020-08-06 15:34:03
#> 94 20-00514119 SW-Dirty Alley Phone 2020-08-06 17:35:16
#> 95 20-00537804 SW-Dirty Alley Phone 2020-08-13 12:24:51
#> 96 20-00591674 SW-Dirty Alley Phone 2020-08-28 19:40:12
#> 97 20-00719585 SW-Dirty Alley API 2020-10-21 09:57:04
#> 98 20-00726802 SW-Dirty Alley Phone 2020-10-23 14:32:42
#> 99 20-00727366 SW-Dirty Alley System 2020-10-23 16:50:09
#> 100 20-00620137 SW-Dirty Alley System 2020-09-09 15:15:37
#> 101 20-00654874 SW-Dirty Alley Phone 2020-09-23 13:24:00
#> 102 20-00666611 SW-Dirty Alley Phone 2020-09-28 15:36:16
#> 103 20-00671377 SW-Dirty Alley Phone 2020-09-30 10:41:15
#> 104 20-00737190 SW-Dirty Alley System 2020-10-28 14:04:29
#> 105 20-00737717 SW-Dirty Alley Phone 2020-10-28 15:48:26
#> 106 20-00753204 SW-Dirty Alley Phone 2020-11-04 16:18:19
#> 107 20-00772383 SW-Dirty Alley Phone 2020-11-13 10:22:32
#> 108 20-00781524 SW-Dirty Alley System 2020-11-17 14:55:45
#> 109 20-00792799 SW-Dirty Alley System 2020-11-23 12:28:03
#> 110 20-00795660 SW-Dirty Alley Phone 2020-11-24 11:05:21
#> 111 20-00798839 SW-Dirty Alley Phone 2020-11-25 10:25:36
#> 112 20-00815065 SW-Dirty Alley Phone 2020-12-03 10:54:41
#> 113 20-00823021 SW-Dirty Alley Phone 2020-12-07 11:17:21
#> 114 20-00834142 SW-Dirty Alley Phone 2020-12-10 15:48:34
#> 115 20-00842211 SW-Dirty Alley Phone 2020-12-14 14:54:29
#> 116 20-00842218 SW-Dirty Alley Phone 2020-12-14 14:55:32
#> 117 20-00842367 SW-Dirty Alley System 2020-12-14 15:37:14
#> 118 20-00847742 SW-Dirty Alley Phone 2020-12-16 09:21:20
#> sr_status sr_status_url
#> 1 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00053788
#> 2 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00059271
#> 3 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00051584
#> 4 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00107202
#> 5 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00110903
#> 6 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00115345
#> 7 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00142040
#> 8 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00062237
#> 9 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00139354
#> 10 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00070593
#> 11 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00100906
#> 12 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00153184
#> 13 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00155352
#> 14 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00159174
#> 15 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00172421
#> 16 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00185891
#> 17 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00204853
#> 18 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00215385
#> 19 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00245245
#> 20 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00245269
#> 21 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00248633
#> 22 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00262746
#> 23 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00254836
#> 24 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00279074
#> 25 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00307201
#> 26 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00323235
#> 27 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00357914
#> 28 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383219
#> 29 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383184
#> 30 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383142
#> 31 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383160
#> 32 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383210
#> 33 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00383863
#> 34 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00382017
#> 35 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00396283
#> 36 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00406083
#> 37 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00424359
#> 38 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00456861
#> 39 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00468460
#> 40 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00460319
#> 41 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00498057
#> 42 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00500273
#> 43 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00518225
#> 44 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00526294
#> 45 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00544733
#> 46 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00602168
#> 47 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00653598
#> 48 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00679949
#> 49 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00704816
#> 50 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00742844
#> 51 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00759532
#> 52 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00785583
#> 53 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00775404
#> 54 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00718065
#> 55 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00729023
#> 56 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00720343
#> 57 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00731337
#> 58 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00725990
#> 59 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00725995
#> 60 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00726393
#> 61 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00834537
#> 62 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00853412
#> 63 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00866979
#> 64 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00888749
#> 65 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00896245
#> 66 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00924055
#> 67 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00928778
#> 68 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00941216
#> 69 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00941211
#> 70 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00944073
#> 71 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00948827
#> 72 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00948939
#> 73 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00969415
#> 74 Closed https://balt311.baltimorecity.gov/citizen/requests/21-00975849
#> 75 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00009981
#> 76 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00042742
#> 77 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00108512
#> 78 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00143990
#> 79 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00150539
#> 80 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00186619
#> 81 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00242451
#> 82 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00256369
#> 83 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00256372
#> 84 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00217790
#> 85 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00292887
#> 86 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00378707
#> 87 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00395207
#> 88 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00411382
#> 89 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00411388
#> 90 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00398279
#> 91 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00401125
#> 92 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00491791
#> 93 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00513600
#> 94 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00514119
#> 95 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00537804
#> 96 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00591674
#> 97 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00719585
#> 98 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00726802
#> 99 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00727366
#> 100 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00620137
#> 101 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00654874
#> 102 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00666611
#> 103 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00671377
#> 104 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00737190
#> 105 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00737717
#> 106 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00753204
#> 107 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00772383
#> 108 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00781524
#> 109 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00792799
#> 110 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00795660
#> 111 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00798839
#> 112 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00815065
#> 113 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00823021
#> 114 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00834142
#> 115 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00842211
#> 116 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00842218
#> 117 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00842367
#> 118 Closed https://balt311.baltimorecity.gov/citizen/requests/20-00847742
#> status_date due_date close_date
#> 1 2021-01-29 15:01:00 2021-02-02 08:07:54 2021-01-29 15:01:21
#> 2 2021-02-03 14:12:00 2021-02-02 16:54:01 2021-02-03 14:12:54
#> 3 2021-01-22 14:34:00 2021-02-01 13:36:09 2021-01-22 14:34:27
#> 4 2021-02-23 14:24:00 2021-02-24 08:57:40 2021-02-23 14:25:02
#> 5 2021-02-16 15:24:00 2021-02-24 10:44:23 2021-02-16 15:24:58
#> 6 2021-02-23 14:26:00 2021-02-24 11:46:25 2021-02-23 14:27:20
#> 7 2021-03-04 15:18:00 2021-03-05 14:37:26 2021-03-04 15:18:37
#> 8 2021-01-25 15:55:00 2021-02-03 15:55:46 2021-01-25 15:56:15
#> 9 2021-03-03 14:21:00 2021-03-04 22:06:52 2021-03-03 14:21:41
#> 10 2021-02-04 15:51:00 2021-02-08 13:57:46 2021-02-04 15:51:52
#> 11 2021-02-23 14:30:00 2021-02-22 08:01:58 2021-02-23 14:30:54
#> 12 2021-03-03 14:22:00 2021-03-09 12:44:37 2021-03-03 14:22:28
#> 13 2021-03-05 14:24:00 2021-03-10 12:38:49 2021-03-05 14:24:34
#> 14 2021-03-05 14:19:00 2021-03-11 13:45:11 2021-03-05 14:19:21
#> 15 2021-03-15 14:51:00 2021-03-16 17:09:48 2021-03-15 14:52:09
#> 16 2021-04-05 16:08:00 2021-03-22 17:08:05 2021-04-05 16:09:32
#> 17 2021-04-06 14:41:00 2021-03-29 17:11:50 2021-04-06 14:41:19
#> 18 2021-04-14 14:11:00 2021-04-01 11:48:32 2021-04-14 14:11:13
#> 19 2021-05-06 13:54:00 2021-04-13 17:09:57 2021-05-06 13:54:11
#> 20 2021-05-06 13:54:00 2021-04-13 17:21:35 2021-05-06 13:54:43
#> 21 2021-05-07 15:16:00 2021-04-14 16:20:02 2021-05-07 15:17:17
#> 22 2021-04-29 15:46:00 2021-04-20 16:27:50 2021-04-29 15:46:19
#> 23 2021-04-29 15:45:00 2021-04-16 13:28:43 2021-04-29 15:45:29
#> 24 2021-04-26 15:15:00 2021-04-26 16:01:25 2021-04-26 15:16:50
#> 25 2021-04-29 15:55:00 2021-05-05 13:55:36 2021-04-29 15:55:23
#> 26 2021-05-07 15:17:00 2021-05-11 09:30:43 2021-05-07 15:17:50
#> 27 2021-05-20 15:17:00 2021-05-24 12:31:49 2021-05-20 15:17:31
#> 28 2021-05-21 15:16:00 2021-06-02 15:15:30 2021-05-21 15:16:17
#> 29 2021-05-21 15:13:00 2021-06-02 15:12:51 2021-05-21 15:13:44
#> 30 2021-05-21 15:09:00 2021-06-02 15:09:15 2021-05-21 15:10:04
#> 31 2021-05-21 15:12:00 2021-06-02 15:10:28 2021-05-21 15:12:28
#> 32 2021-05-21 15:14:00 2021-06-02 15:14:26 2021-05-21 15:15:06
#> 33 2021-05-25 14:27:00 2021-06-02 19:21:21 2021-05-25 14:27:10
#> 34 2021-05-25 14:26:00 2021-06-02 11:12:16 2021-05-25 14:26:36
#> 35 2021-06-02 14:32:00 2021-06-07 15:05:24 2021-06-02 14:32:43
#> 36 2021-06-02 14:43:00 2021-06-09 12:44:57 2021-06-02 14:43:22
#> 37 2021-06-14 15:10:00 2021-06-15 13:09:03 2021-06-14 15:10:39
#> 38 2021-06-23 13:56:00 2021-06-28 11:19:24 2021-06-23 13:56:54
#> 39 2021-06-30 14:51:00 2021-07-01 11:44:24 2021-06-30 14:54:57
#> 40 2021-06-22 15:21:00 2021-06-29 13:11:34 2021-06-22 15:22:04
#> 41 2021-07-08 14:51:00 2021-07-14 11:04:06 2021-07-08 14:51:55
#> 42 2021-07-06 15:32:00 2021-07-14 18:48:40 2021-07-06 15:33:18
#> 43 2021-07-20 14:16:00 2021-07-20 15:28:32 2021-07-20 14:16:23
#> 44 2021-07-27 15:15:00 2021-07-22 11:11:08 2021-07-27 15:15:48
#> 45 2021-07-27 15:16:00 2021-07-28 17:37:31 2021-07-27 15:16:17
#> 46 2021-08-16 15:11:00 2021-08-18 14:43:24 2021-08-16 15:11:59
#> 47 2021-09-02 15:04:00 2021-09-07 15:57:44 2021-09-02 15:04:23
#> 48 2021-09-11 14:13:00 2021-09-15 07:29:58 2021-09-11 14:13:39
#> 49 2021-09-15 15:07:00 2021-09-24 11:44:24 2021-09-15 15:07:56
#> 50 2021-09-29 14:47:00 2021-10-07 18:49:15 2021-09-29 14:48:09
#> 51 2021-10-12 14:48:00 2021-10-13 13:05:36 2021-10-12 14:48:50
#> 52 2021-10-20 15:15:00 2021-10-20 10:52:18 2021-10-20 15:15:41
#> 53 2021-10-12 14:45:00 2021-10-19 09:35:42 2021-10-12 14:45:52
#> 54 2021-09-22 14:59:00 2021-09-29 10:06:08 2021-09-22 14:59:40
#> 55 2021-09-24 15:00:00 2021-10-04 12:37:19 2021-09-24 15:01:03
#> 56 2021-09-21 14:25:00 2021-09-29 19:26:57 2021-09-21 14:26:12
#> 57 2021-09-25 15:52:00 2021-10-05 09:41:58 2021-09-25 15:52:18
#> 58 2021-09-24 15:02:00 2021-10-01 13:00:02 2021-09-24 15:03:13
#> 59 2021-09-24 15:03:00 2021-10-01 13:01:29 2021-09-24 15:03:45
#> 60 2021-09-24 15:01:00 2021-10-01 14:46:02 2021-09-24 15:01:42
#> 61 2021-11-02 14:43:00 2021-11-08 12:40:39 2021-11-02 14:44:25
#> 62 2021-11-05 13:51:00 2021-11-16 14:15:30 2021-11-05 13:51:16
#> 63 2021-11-10 14:45:00 2021-11-19 16:07:05 2021-11-10 14:45:23
#> 64 2021-11-18 14:44:00 2021-11-29 14:57:40 2021-11-18 14:45:16
#> 65 2021-11-23 15:12:00 2021-12-01 11:44:50 2021-11-23 15:12:28
#> 66 2021-12-04 07:25:00 2021-12-13 15:38:46 2021-12-04 07:25:39
#> 67 2021-12-06 15:01:00 2021-12-14 13:01:24 2021-12-06 15:01:36
#> 68 2021-12-09 14:36:00 2021-12-17 14:50:21 2021-12-09 14:40:52
#> 69 2021-12-09 14:20:00 2021-12-17 14:49:14 2021-12-09 14:40:59
#> 70 2021-12-10 15:15:00 2021-12-20 13:50:59 2021-12-10 15:15:19
#> 71 2021-12-13 14:33:00 2021-12-21 09:53:47 2021-12-13 14:33:56
#> 72 2021-12-13 14:34:00 2021-12-21 10:54:17 2021-12-13 14:34:42
#> 73 2021-12-21 14:44:00 2021-12-30 08:44:51 2021-12-21 14:48:59
#> 74 2021-12-23 15:09:00 2022-01-04 13:46:20 2021-12-23 15:09:37
#> 75 2020-01-11 16:35:38 2020-01-15 22:34:50 2020-01-11 16:35:39
#> 76 2020-01-31 13:45:21 2020-02-03 14:19:56 2020-01-31 13:45:22
#> 77 2020-03-02 15:28:45 2020-03-03 18:13:18 2020-03-02 15:27:47
#> 78 2020-03-13 14:18:42 2020-03-20 10:04:25 2020-03-13 14:18:44
#> 79 2020-03-17 15:52:04 2020-03-24 14:26:56 2020-03-17 15:52:05
#> 80 2020-05-07 15:12:57 2020-04-02 10:56:29 2020-05-07 15:12:58
#> 81 2020-06-03 15:18:37 2020-05-07 13:29:54 2020-06-03 15:18:39
#> 82 2020-05-12 14:40:51 2020-05-15 10:43:39 2020-05-12 14:40:52
#> 83 2020-05-12 14:42:03 2020-05-15 10:45:17 2020-05-12 14:42:04
#> 84 2020-05-12 14:39:54 2020-04-22 09:58:47 2020-05-12 14:39:56
#> 85 2020-07-06 14:30:11 2020-06-05 13:56:24 2020-07-06 14:30:12
#> 86 2020-07-09 14:26:35 2020-07-02 13:56:29 2020-07-09 14:26:37
#> 87 2020-07-22 14:04:10 2020-07-09 14:55:17 2020-07-22 14:04:12
#> 88 2020-07-28 15:20:13 2020-07-14 16:34:24 2020-07-28 15:20:14
#> 89 2020-07-06 08:33:14 2020-07-14 16:34:56 2020-07-06 08:33:15
#> 90 2020-07-21 14:34:42 2020-07-10 12:24:50 2020-07-21 14:34:43
#> 91 2020-07-22 14:06:08 2020-07-13 08:47:37 2020-07-22 14:06:10
#> 92 2020-08-17 14:33:21 2020-08-10 15:23:40 2020-08-17 14:33:22
#> 93 2020-08-07 15:06:28 2020-08-17 15:34:03 2020-08-07 15:06:29
#> 94 2020-10-07 15:54:42 2020-08-17 17:35:16 2020-10-07 15:54:44
#> 95 2020-10-05 14:32:42 2020-08-24 12:24:51 2020-10-05 14:32:44
#> 96 2020-10-08 15:38:37 2020-09-09 19:40:12 2020-10-08 15:38:39
#> 97 2020-10-22 13:55:40 2020-10-30 09:57:04 2020-10-22 13:55:42
#> 98 2020-11-04 15:30:19 2020-11-04 13:32:42 2020-11-04 15:30:20
#> 99 2020-10-26 14:29:58 2020-11-04 15:50:09 2020-10-26 14:30:00
#> 100 2020-10-05 14:33:16 2020-09-18 15:15:37 2020-10-05 14:33:18
#> 101 2020-10-08 15:39:19 2020-10-02 13:23:59 2020-10-08 15:39:20
#> 102 2020-10-07 14:40:03 2020-10-07 15:36:16 2020-10-07 14:40:05
#> 103 2020-10-08 15:30:36 2020-10-09 10:41:15 2020-10-08 15:30:37
#> 104 2020-10-29 14:20:42 2020-11-09 13:04:29 2020-10-29 14:20:44
#> 105 2020-10-29 14:11:41 2020-11-09 14:48:26 2020-10-29 14:11:43
#> 106 2020-11-17 14:02:48 2020-11-16 16:18:18 2020-11-17 14:02:51
#> 107 2020-11-17 14:04:08 2020-11-25 10:22:32 2020-11-17 14:04:10
#> 108 2020-11-19 14:46:23 2020-11-27 14:55:45 2020-11-19 14:46:24
#> 109 2020-11-24 14:38:59 2020-12-02 12:28:03 2020-11-24 14:39:01
#> 110 2020-11-27 15:09:02 2020-12-03 11:05:21 2020-11-27 15:09:04
#> 111 2020-11-27 15:09:40 2020-12-04 10:25:36 2020-11-27 15:09:41
#> 112 2020-12-04 14:43:37 2020-12-14 10:54:41 2020-12-04 14:43:39
#> 113 2020-12-08 15:46:44 2020-12-16 11:17:21 2020-12-08 15:46:45
#> 114 2020-12-15 14:40:24 2020-12-21 15:48:34 2020-12-15 14:40:25
#> 115 2020-12-15 15:33:32 2020-12-23 14:54:29 2020-12-15 15:33:34
#> 116 2020-12-15 15:34:17 2020-12-23 14:55:32 2020-12-15 15:34:19
#> 117 2020-12-15 15:32:30 2020-12-23 15:37:14 2020-12-15 15:32:31
#> 118 2020-12-18 14:44:44 2020-12-28 09:21:20 2020-12-18 14:44:46
#> agency last_activity
#> 1 Solid Waste Service Response
#> 2 Solid Waste Service Response
#> 3 Solid Waste Service Response
#> 4 Solid Waste Service Response
#> 5 Solid Waste Service Response
#> 6 Solid Waste Service Response
#> 7 Solid Waste Service Response
#> 8 Solid Waste Service Response
#> 9 Solid Waste Service Response
#> 10 Solid Waste Service Response
#> 11 Solid Waste Service Response
#> 12 Solid Waste Service Response
#> 13 Solid Waste Service Response
#> 14 Solid Waste Service Response
#> 15 Solid Waste Service Response
#> 16 Solid Waste Service Response
#> 17 Solid Waste Service Response
#> 18 Solid Waste Service Response
#> 19 Solid Waste Service Response
#> 20 Solid Waste Service Response
#> 21 Solid Waste Service Response
#> 22 Solid Waste Service Response
#> 23 Solid Waste Service Response
#> 24 Solid Waste Service Response
#> 25 Solid Waste Service Response
#> 26 Solid Waste Service Response
#> 27 Solid Waste Service Response
#> 28 Solid Waste Service Response
#> 29 Solid Waste Service Response
#> 30 Solid Waste Service Response
#> 31 Solid Waste Service Response
#> 32 Solid Waste Service Response
#> 33 Solid Waste Service Response
#> 34 Solid Waste Service Response
#> 35 Solid Waste Service Response
#> 36 Solid Waste Service Response
#> 37 Solid Waste Service Response
#> 38 Solid Waste Service Response
#> 39 Solid Waste Service Response
#> 40 Solid Waste Service Response
#> 41 Solid Waste Service Response
#> 42 Solid Waste Service Response
#> 43 Solid Waste Service Response
#> 44 Solid Waste Service Response
#> 45 Solid Waste Service Response
#> 46 Solid Waste Service Response
#> 47 Solid Waste Service Response
#> 48 Solid Waste Service Response
#> 49 Solid Waste Service Response
#> 50 Solid Waste Service Response
#> 51 Solid Waste Service Response
#> 52 Solid Waste Service Response
#> 53 Solid Waste Service Response
#> 54 Solid Waste Service Response
#> 55 Solid Waste Service Response
#> 56 Solid Waste Service Response
#> 57 Solid Waste Service Response
#> 58 Solid Waste Service Response
#> 59 Solid Waste Service Response
#> 60 Solid Waste Service Response
#> 61 Solid Waste Service Response
#> 62 Solid Waste Service Response
#> 63 Solid Waste Service Response
#> 64 Solid Waste Service Response
#> 65 Solid Waste Service Response
#> 66 Solid Waste Service Response
#> 67 Solid Waste Service Response
#> 68 Solid Waste Service Response
#> 69 Solid Waste Service Response
#> 70 Solid Waste Service Response
#> 71 Solid Waste Service Response
#> 72 Solid Waste Service Response
#> 73 Solid Waste Service Response
#> 74 Solid Waste Service Response
#> 75 Solid Waste Service Response
#> 76 Solid Waste Service Response
#> 77 Solid Waste Service Response
#> 78 Solid Waste Service Response
#> 79 Solid Waste Service Response
#> 80 Solid Waste Service Response
#> 81 Solid Waste Service Response
#> 82 Solid Waste Service Response
#> 83 Solid Waste Service Response
#> 84 Solid Waste Service Response
#> 85 Solid Waste Service Response
#> 86 Solid Waste Service Response
#> 87 Solid Waste Service Response
#> 88 Solid Waste Service Response
#> 89 Solid Waste Service Response
#> 90 Solid Waste Service Response
#> 91 Solid Waste Service Response
#> 92 Solid Waste Service Response
#> 93 Solid Waste Service Response
#> 94 Solid Waste Service Response
#> 95 Solid Waste Service Response
#> 96 Solid Waste Service Response
#> 97 Solid Waste Service Response
#> 98 Solid Waste Service Response
#> 99 Solid Waste Service Response
#> 100 Solid Waste Service Response
#> 101 Solid Waste Service Response
#> 102 Solid Waste Service Response
#> 103 Solid Waste Service Response
#> 104 Solid Waste Service Response
#> 105 Solid Waste Service Response
#> 106 Solid Waste Service Response
#> 107 Solid Waste Service Response
#> 108 Solid Waste Service Response
#> 109 Solid Waste Service Response
#> 110 Solid Waste Service Response
#> 111 Solid Waste Service Response
#> 112 Solid Waste Service Response
#> 113 Solid Waste Service Response
#> 114 Solid Waste Service Response
#> 115 Solid Waste Service Response
#> 116 Solid Waste Service Response
#> 117 Solid Waste Service Response
#> 118 Solid Waste Service Response
#> last_activity_date outcome
#> 1 2021-01-29 15:01:00 Work completed
#> 2 2021-02-03 14:12:00 Work completed
#> 3 2021-01-22 14:34:00 Work completed
#> 4 2021-02-23 14:24:00 SR assessed and no cause for action determined
#> 5 2021-02-16 15:24:00 SR assessed and no cause for action determined
#> 6 2021-02-23 14:26:00 SR assessed and no cause for action determined
#> 7 2021-03-04 15:18:00 Work completed
#> 8 2021-01-25 15:55:00 Work completed
#> 9 2021-03-03 14:21:00 SR assessed and no cause for action determined
#> 10 2021-02-04 15:51:00 Work completed
#> 11 2021-02-23 14:30:00 SR assessed and no cause for action determined
#> 12 2021-03-03 14:22:00 Work completed
#> 13 2021-03-05 14:24:00 SR assessed and no cause for action determined
#> 14 2021-03-05 14:19:00 Work completed
#> 15 2021-03-15 14:51:00 Work completed
#> 16 2021-04-05 16:08:00 Work completed
#> 17 2021-04-06 14:41:00 Work completed
#> 18 2021-04-14 14:11:00 Work completed
#> 19 2021-05-06 13:54:00 Work completed
#> 20 2021-05-06 13:54:00 Work completed
#> 21 2021-05-07 15:16:00 SR assessed and no cause for action determined
#> 22 2021-04-29 15:46:00 SR assessed and no cause for action determined
#> 23 2021-04-29 15:45:00 SR assessed and no cause for action determined
#> 24 2021-04-26 15:15:00 Work completed
#> 25 2021-04-29 15:55:00 Work completed
#> 26 2021-05-07 15:17:00 Work completed
#> 27 2021-05-20 15:17:00 Work completed
#> 28 2021-05-21 15:16:00 Work completed
#> 29 2021-05-21 15:13:00 Work completed
#> 30 2021-05-21 15:09:00 Work completed
#> 31 2021-05-21 15:12:00 Work completed
#> 32 2021-05-21 15:14:00 Work completed
#> 33 2021-05-25 14:27:00 Work completed
#> 34 2021-05-25 14:26:00 Work completed
#> 35 2021-06-02 14:32:00 Work completed
#> 36 2021-06-02 14:43:00 Work completed
#> 37 2021-06-14 15:10:00 SR assessed and no cause for action determined
#> 38 2021-06-23 13:56:00 Work completed
#> 39 2021-06-30 14:51:00 SR assessed and no cause for action determined
#> 40 2021-06-22 15:21:00 Work completed
#> 41 2021-07-08 14:51:00 Work completed
#> 42 2021-07-06 15:32:00 SR assessed and no cause for action determined
#> 43 2021-07-20 14:16:00 Work completed
#> 44 2021-07-27 15:15:00 Work completed
#> 45 2021-07-27 15:16:00 Work completed
#> 46 2021-08-16 15:11:00 Work completed
#> 47 2021-09-02 15:04:00 Work completed
#> 48 2021-09-11 14:13:00 Work completed
#> 49 2021-09-15 15:07:00 Work completed
#> 50 2021-09-29 14:47:00 Work completed
#> 51 2021-10-12 14:48:00 Work completed
#> 52 2021-10-20 15:15:00 Work completed
#> 53 2021-10-12 14:45:00 Work completed
#> 54 2021-09-22 14:59:00 SR assessed and no cause for action determined
#> 55 2021-09-24 15:00:00 Work completed
#> 56 2021-09-21 14:25:00 Work completed
#> 57 2021-09-25 15:52:00 SR assessed and no cause for action determined
#> 58 2021-09-24 15:02:00 SR assessed and no cause for action determined
#> 59 2021-09-24 15:03:00 Work completed
#> 60 2021-09-24 15:01:00 Work completed
#> 61 2021-11-02 14:43:00 Work completed
#> 62 2021-11-05 13:51:00 Work completed
#> 63 2021-11-10 14:45:00 Work completed
#> 64 2021-11-18 14:44:00 SR assessed and no cause for action determined
#> 65 2021-11-23 15:12:00 Work completed
#> 66 2021-12-04 07:25:00 SR assessed and no cause for action determined
#> 67 2021-12-06 15:01:00 Work completed
#> 68 2021-12-09 14:36:00 Work completed
#> 69 2021-12-09 14:20:00 Work completed
#> 70 2021-12-10 15:15:00 Work completed
#> 71 2021-12-13 14:33:00 SR assessed and no cause for action determined
#> 72 2021-12-13 14:34:00 SR assessed and no cause for action determined
#> 73 2021-12-21 14:44:00 SR assessed and no cause for action determined
#> 74 2021-12-23 15:09:00 Work completed
#> 75 2020-01-11 16:34:00 Work completed
#> 76 2020-01-31 13:44:00 Work completed
#> 77 2020-03-02 15:27:00 SR assessed and no cause for action determined
#> 78 2020-03-13 14:18:00 Work completed
#> 79 2020-03-17 15:51:00 Work completed
#> 80 2020-05-07 15:12:00 Work completed
#> 81 2020-06-03 15:18:00 Work completed
#> 82 2020-05-12 14:40:00 Work completed
#> 83 2020-05-12 14:41:00 Work completed
#> 84 2020-05-12 14:39:00 Work completed
#> 85 2020-07-06 14:29:00 SR assessed and no cause for action determined
#> 86 2020-07-09 14:26:00 Work completed
#> 87 2020-07-22 14:01:00 Work completed
#> 88 2020-07-28 15:19:00 Work completed
#> 89 2020-07-06 08:32:00 Work completed
#> 90 2020-07-21 14:33:00 Work completed
#> 91 2020-07-22 14:05:00 Work completed
#> 92 2020-08-17 14:33:00 Work completed
#> 93 2020-08-07 15:03:00 SR assessed and no cause for action determined
#> 94 2020-10-07 15:54:00 Work completed
#> 95 2020-10-05 14:32:00 SR assessed and no cause for action determined
#> 96 2020-10-08 15:38:00 Work completed
#> 97 2020-10-22 13:55:00 Work completed
#> 98 2020-11-04 15:30:00 Work completed
#> 99 2020-10-26 14:29:00 Work completed
#> 100 2020-10-05 14:33:00 SR assessed and no cause for action determined
#> 101 2020-10-08 15:39:00 Work completed
#> 102 2020-10-07 14:39:00 Work completed
#> 103 2020-10-08 15:30:00 Work completed
#> 104 2020-10-29 14:20:00 SR assessed and no cause for action determined
#> 105 2020-10-29 14:11:00 Work completed
#> 106 2020-11-17 14:02:00 Work completed
#> 107 2020-11-17 14:03:00 SR assessed and no cause for action determined
#> 108 2020-11-19 14:46:00 Work completed
#> 109 2020-11-24 14:38:00 Work completed
#> 110 2020-11-27 15:08:00 Work completed
#> 111 2020-11-27 15:09:00 Work completed
#> 112 2020-12-04 14:43:00 Work completed
#> 113 2020-12-08 15:46:00 SR assessed and no cause for action determined
#> 114 2020-12-15 14:40:00 Work completed
#> 115 2020-12-15 15:33:00 Work completed
#> 116 2020-12-15 15:34:00 Work completed
#> 117 2020-12-15 15:32:00 Work completed
#> 118 2020-12-18 14:44:00 SR assessed and no cause for action determined
#> days_to_close address zip_code neighborhood
#> 1 7.29 800 WILDWOOD PKWY 21229 Edmondson Village
#> 2 9.89 826 N WOODINGTON RD 21229 Rognel Heights
#> 3 1.04 905 MOUNT HOLLY ST 21229 Edgewood
#> 4 11.23 4000 COLBORNE RD 21229 Edmondson Village
#> 5 3.19 3801 ROKEBY RD 21229 Edmondson Village
#> 6 8.11 4009 COLBORNE RD 21229 Edmondson Village
#> 7 8.03 631 N WOODINGTON RD 21229 Edmondson Village
#> 8 0.00 704 MOUNT HOLLY ST 21229 Edmondson Village
#> 9 7.68 4010 GELSTON DR 21229 Edmondson Village
#> 10 7.08 3909 COLBORNE RD 21229 Edmondson Village
#> 11 13.27 901 MOUNT HOLLY ST 21229 Edgewood
#> 12 3.07 3909 COLBORNE RD 21229 Edmondson Village
#> 13 4.07 731 MOUNT HOLLY ST 21229 Edgewood
#> 14 3.02 1105 LYNDHURST ST 21229 Edmondson Village
#> 15 7.95 3909 COLBORNE RD 21229 Edmondson Village
#> 16 25.00 800 N WOODINGTON RD 21229 Rognel Heights
#> 17 18.90 3900 ROKEBY RD 21229 Edmondson Village
#> 18 22.10 725 MOUNT HOLLY ST 21229 Edgewood
#> 19 32.86 3908 WOODRIDGE RD 21229 Edmondson Village
#> 20 32.86 3901 COLBORNE RD 21229 Edmondson Village
#> 21 31.96 608 N WOODINGTON RD 21229 Rognel Heights
#> 22 19.97 800 KEVIN RD 21229 Rognel Heights
#> 23 22.09 816 WILDWOOD PKWY 21229 Edmondson Village
#> 24 10.97 4006 WOODRIDGE RD 21229 Edmondson Village
#> 25 3.08 801 KEVIN RD 21229 Rognel Heights
#> 26 6.24 829 N AUGUSTA AVE 21229 Edmondson Village
#> 27 7.12 3924 CRANSTON AVE 21229 Edmondson Village
#> 28 0.00 3909 ROKEBY RD 21229 Edmondson Village
#> 29 0.00 1008 LYNDHURST ST 21229 Edmondson Village
#> 30 0.00 1100 WILDWOOD PKWY 21229 Edmondson Village
#> 31 0.00 1206 WILDWOOD PKWY 21229 Edmondson Village
#> 32 0.00 3902 FLOWERTON RD 21229 Edmondson Village
#> 33 3.80 4000 COLBORNE RD 21229 Edmondson Village
#> 34 4.13 3916 GELSTON DR 21229 Edmondson Village
#> 35 6.98 1200 BLK N AUGUSTA-MOUNTWOOD 21229 Edmondson Village
#> 36 3.08 1000 N WOODINGTON RD 21229 Rognel Heights
#> 37 8.08 1248 N AUGUSTA AVE 21229 Edmondson Village
#> 38 6.11 3801 STOKES DR 21229 Edmondson Village
#> 39 8.13 3810 GELSTON DR 21229 Edmondson Village
#> 40 4.09 4022 CRANSTON AVE 21229 Edmondson Village
#> 41 6.16 FLOWERTON RD & N WOODINGTON RD 21229 Rognel Heights
#> 42 3.86 3810 GELSTON DR 21229 Edmondson Village
#> 43 10.95 813 N AUGUSTA AVE 21229 Edmondson Village
#> 44 14.17 3814 GELCRAN LN 21229 Edmondson Village
#> 45 7.90 4021 CRANSTON AVE 21229 Edmondson Village
#> 46 7.02 3809 STOKES DR 21229 Edmondson Village
#> 47 6.96 901 N WOODINGTON RD 21229 Edmondson Village
#> 48 5.28 3902 WOODRIDGE RD 21229 Edmondson Village
#> 49 0.14 3919 FLOWERTON RD 21229 Edmondson Village
#> 50 0.83 600 WILDWOOD PKWY 21229 Edmondson Village
#> 51 11.07 3900 EDMONDSON AVE 21229 Edmondson Village
#> 52 9.18 3819 CRANSTON AVE 21229 Edmondson Village
#> 53 5.22 3900 EDMONDSON AVE 21229 Edmondson Village
#> 54 2.20 1252 N AUGUSTA AVE 21229 Edmondson Village
#> 55 1.10 600 N AUGUSTA AVE 21229 Edmondson Village
#> 56 0.79 601 N LOUDON AVE 21229 Edmondson Village
#> 57 1.26 3800 GELSTON DR 21229 Edmondson Village
#> 58 2.09 3901 FLOWERTON RD 21229 Edmondson Village
#> 59 2.08 3900 FLOWERTON RD 21229 Edmondson Village
#> 60 2.01 711 LYNDHURST ST 21229 Edmondson Village
#> 61 5.04 3909 COLBORNE RD 21229 Edmondson Village
#> 62 0.94 3807 STOKES DR 21229 Edmondson Village
#> 63 0.94 1106 LYNDHURST ST 21229 Edmondson Village
#> 64 0.99 4100 GLEN HUNT RD 21229 Edmondson Village
#> 65 3.14 4100 GLEN HUNT RD 21229 Edmondson Village
#> 66 1.66 3900 EDMONDSON AVE 21229 Edmondson Village
#> 67 2.08 4201 GELSTON DR 21229 Rognel Heights
#> 68 0.99 700 BLK LYNDHURST ST 21229 Edmondson Village
#> 69 0.99 3814 CRANSTON AVE 21229 Edmondson Village
#> 70 1.06 700 WICKLOW RD 21229 Rognel Heights
#> 71 2.19 913 LYNDHURST ST 21229 Edmondson Village
#> 72 2.15 3900 EDMONDSON AVE 21229 Edmondson Village
#> 73 1.25 913 LYNDHURST ST 21229 Edmondson Village
#> 74 1.06 3807 STOKES DR 21229 Edmondson Village
#> 75 4.75 3909 COLBORNE RD 21229 Edmondson Village
#> 76 7.98 3909 COLBORNE RD 21229 Edmondson Village
#> 77 7.89 4225 COLBORNE RD 21229 Rognel Heights
#> 78 2.18 712 WICKLOW RD 21229 Rognel Heights
#> 79 4.06 600 BLK WICKLOW RD 21229 Rognel Heights
#> 80 44.18 600 BLK WICKLOW RD 21229 Rognel Heights
#> 81 36.08 4102 WOODRIDGE RD 21229 Edmondson Village
#> 82 6.16 3900 FLOWERTON RD 21229 Edmondson Village
#> 83 6.16 3900 ROKEBY RD 21229 Edmondson Village
#> 84 29.20 723 MOUNT HOLLY ST 21229 Edgewood
#> 85 41.02 1008 WILDWOOD PKWY 21229 Edmondson Village
#> 86 16.02 4105 FLOWERTON RD 21229 Edmondson Village
#> 87 22.96 601 N WOODINGTON RD 21229 Edmondson Village
#> 88 24.97 1100 LYNDHURST ST 21229 Edmondson Village
#> 89 2.69 1100 LYNDHURST ST 21229 Edmondson Village
#> 90 21.09 4003 COLBORNE RD 21229 Edmondson Village
#> 91 21.22 622 WICKLOW RD 21229 Rognel Heights
#> 92 17.97 4029 CRANSTON AVE 21229 Edmondson Village
#> 93 0.98 622 WICKLOW RD 21229 Rognel Heights
#> 94 61.93 4100 MOUNTWOOD RD 21229 Edmondson Village
#> 95 53.09 4200 FLOWERTON RD 21229 Rognel Heights
#> 96 40.83 4000 WOODRIDGE RD 21229 Edmondson Village
#> 97 1.17 1102 MOUNT HOLLY ST 21229 Edmondson Village
#> 98 12.04 1000 MOUNT HOLLY ST 21229 Edmondson Village
#> 99 2.90 4200 EDMONDSON AVE 21229 Rognel Heights
#> 100 25.97 700 WILDWOOD PKWY 21229 Edmondson Village
#> 101 15.09 3930 ROKEBY RD 21229 Edmondson Village
#> 102 8.96 3901 COLBORNE RD 21229 Edmondson Village
#> 103 8.20 3815 HARLEM AVE 21229 Edmondson Village
#> 104 1.01 718 LYNDHURST ST 21229 Edmondson Village
#> 105 0.93 1011 MOUNT HOLLY ST 21229 Edgewood
#> 106 12.91 3901 COLBORNE RD 21229 Edmondson Village
#> 107 4.15 600 MOUNT HOLLY ST 21229 Edmondson Village
#> 108 1.99 3901 COLBORNE RD 21229 Edmondson Village
#> 109 1.09 3901 COLBORNE RD 21229 Edmondson Village
#> 110 3.17 718 LYNDHURST ST 21229 Edmondson Village
#> 111 2.20 4009 COLBORNE RD 21229 Edmondson Village
#> 112 1.16 618 WICKLOW RD 21229 Rognel Heights
#> 113 1.19 3901 WOODRIDGE RD 21229 Edmondson Village
#> 114 4.95 3901 COLBORNE RD 21229 Edmondson Village
#> 115 1.03 4105 MOUNTWOOD RD 21229 Edmondson Village
#> 116 1.03 4107 MOUNTWOOD RD 21229 Edmondson Village
#> 117 1.00 602 N WOODINGTON RD 21229 Rognel Heights
#> 118 2.22 4009 COLBORNE RD 21229 Edmondson Village
#> council_district police_district latitude longitude
#> 1 8 Southwestern 39.29641 -76.68416
#> 2 8 Southwestern 39.29722 -76.68637
#> 3 8 Southwestern 39.29815 -76.67980
#> 4 8 Southwestern 39.29801 -76.68264
#> 5 8 Southwestern 39.29923 -76.68060
#> 6 8 Southwestern 39.29766 -76.68282
#> 7 8 Southwestern 39.29505 -76.68574
#> 8 8 Southwestern 39.29535 -76.67989
#> 9 8 Southwestern 39.29579 -76.68288
#> 10 8 Southwestern 39.29768 -76.68213
#> 11 8 Southwestern 39.29804 -76.67980
#> 12 8 Southwestern 39.29768 -76.68213
#> 13 8 Southwestern 39.29581 -76.67985
#> 14 8 Southwestern 39.29963 -76.68127
#> 15 8 Southwestern 39.29768 -76.68213
#> 16 8 Southwestern 39.29633 -76.68635
#> 17 8 Southwestern 39.29954 -76.68205
#> 18 8 Southwestern 39.29576 -76.67985
#> 19 8 Southwestern 39.29729 -76.68211
#> 20 8 Southwestern 39.29769 -76.68193
#> 21 8 Southwestern 39.29437 -76.68622
#> 22 8 Southwestern 39.29634 -76.68734
#> 23 8 Southwestern 39.29686 -76.68412
#> 24 8 Southwestern 39.29727 -76.68279
#> 25 8 Southwestern 39.29661 -76.68706
#> 26 8 Southwestern 39.29729 -76.68491
#> 27 8 Southwestern 39.29656 -76.68246
#> 28 8 Southwestern 39.29918 -76.68228
#> 29 8 Southwestern 39.29893 -76.68169
#> 30 8 Southwestern 39.29944 -76.68407
#> 31 8 Southwestern 39.30028 -76.68406
#> 32 8 Southwestern 39.29877 -76.68205
#> 33 8 Southwestern 39.29801 -76.68264
#> 34 8 Southwestern 39.29581 -76.68224
#> 35 8 Southwestern 39.30085 -76.68512
#> 36 8 Southwestern 39.29855 -76.68644
#> 37 8 Southwestern 39.30055 -76.68549
#> 38 8 Southwestern 39.29983 -76.68057
#> 39 8 Southwestern 39.29578 -76.68062
#> 40 8 Southwestern 39.29653 -76.68315
#> 41 8 Southwestern 39.29846 -76.68619
#> 42 8 Southwestern 39.29578 -76.68062
#> 43 8 Southwestern 39.29670 -76.68488
#> 44 8 Southwestern 39.29601 -76.68068
#> 45 8 Southwestern 39.29618 -76.68309
#> 46 8 Southwestern 39.29997 -76.68081
#> 47 8 Southwestern 39.29782 -76.68590
#> 48 8 Southwestern 39.29729 -76.68196
#> 49 8 Southwestern 39.29842 -76.68258
#> 50 8 Southwestern 39.29419 -76.68428
#> 51 8 Southwestern 39.29410 -76.68137
#> 52 8 Southwestern 39.29626 -76.68085
#> 53 8 Southwestern 39.29410 -76.68137
#> 54 8 Southwestern 39.30104 -76.68600
#> 55 8 Southwestern 39.29416 -76.68524
#> 56 8 Southwestern 39.29425 -76.68232
#> 57 8 Southwestern 39.29575 -76.68034
#> 58 8 Southwestern 39.29844 -76.68198
#> 59 8 Southwestern 39.29877 -76.68199
#> 60 8 Southwestern 39.29550 -76.68104
#> 61 8 Southwestern 39.29768 -76.68213
#> 62 8 Southwestern 39.29999 -76.68051
#> 63 8 Southwestern 39.29968 -76.68173
#> 64 8 Southwestern 39.30014 -76.68434
#> 65 8 Southwestern 39.30014 -76.68434
#> 66 8 Southwestern 39.29410 -76.68137
#> 67 8 Southwestern 39.29477 -76.68687
#> 68 8 Southwestern 39.29604 -76.68132
#> 69 8 Southwestern 39.29659 -76.68076
#> 70 8 Southwestern 39.29515 -76.68702
#> 71 8 Southwestern 39.29831 -76.68122
#> 72 8 Southwestern 39.29410 -76.68137
#> 73 8 Southwestern 39.29831 -76.68122
#> 74 8 Southwestern 39.29993 -76.68075
#> 75 8 Southwestern 39.29768 -76.68213
#> 76 8 Southwestern 39.29768 -76.68213
#> 77 8 Southwestern 39.29725 -76.68752
#> 78 8 Southwestern 39.29538 -76.68736
#> 79 8 Southwestern 39.29495 -76.68636
#> 80 8 Southwestern 39.29495 -76.68636
#> 81 8 Southwestern 39.29721 -76.68452
#> 82 8 Southwestern 39.29877 -76.68199
#> 83 8 Southwestern 39.29954 -76.68205
#> 84 8 Southwestern 39.29574 -76.67985
#> 85 8 Southwestern 39.29867 -76.68399
#> 86 8 Southwestern 39.29835 -76.68462
#> 87 8 Southwestern 39.29415 -76.68569
#> 88 8 Southwestern 39.29953 -76.68172
#> 89 8 Southwestern 39.29953 -76.68172
#> 90 8 Southwestern 39.29767 -76.68266
#> 91 8 Southwestern 39.29476 -76.68641
#> 92 8 Southwestern 39.29618 -76.68330
#> 93 8 Southwestern 39.29476 -76.68641
#> 94 8 Southwestern 39.30083 -76.68448
#> 95 8 Southwestern 39.29860 -76.68678
#> 96 8 Southwestern 39.29727 -76.68265
#> 97 8 Southwestern 39.29962 -76.68033
#> 98 8 Southwestern 39.29877 -76.68025
#> 99 8 Southwestern 39.29392 -76.68610
#> 100 8 Southwestern 39.29565 -76.68413
#> 101 8 Southwestern 39.29951 -76.68297
#> 102 8 Southwestern 39.29769 -76.68193
#> 103 8 Southwestern 39.29495 -76.68078
#> 104 8 Southwestern 39.29589 -76.68154
#> 105 8 Southwestern 39.29905 -76.67984
#> 106 8 Southwestern 39.29769 -76.68193
#> 107 8 Southwestern 39.29400 -76.67982
#> 108 8 Southwestern 39.29769 -76.68193
#> 109 8 Southwestern 39.29769 -76.68193
#> 110 8 Southwestern 39.29578 -76.68135
#> 111 8 Southwestern 39.29766 -76.68282
#> 112 8 Southwestern 39.29482 -76.68621
#> 113 8 Southwestern 39.29695 -76.68188
#> 114 8 Southwestern 39.29769 -76.68193
#> 115 8 Southwestern 39.30048 -76.68449
#> 116 8 Southwestern 39.30049 -76.68454
#> 117 8 Southwestern 39.29419 -76.68622
#> 118 8 Southwestern 39.29766 -76.68282
#> geometry objectid
#> 1 POINT (427245.1 180958.8) NA
#> 2 POINT (427053.6 181048.6) NA
#> 3 POINT (427619.9 181153.5) NA
#> 4 POINT (427375.7 181136.8) NA
#> 5 POINT (427550.9 181273.1) NA
#> 6 POINT (427359.6 181098.7) NA
#> 7 POINT (427109.2 180807.5) NA
#> 8 POINT (427613.6 180842.2) NA
#> 9 POINT (427355.8 180890.3) NA
#> 10 POINT (427419.8 181100.8) NA
#> 11 POINT (427620.4 181141.4) NA
#> 12 POINT (427419.8 181100.8) NA
#> 13 POINT (427616.5 180893.3) NA
#> 14 POINT (427493.3 181317) NA
#> 15 POINT (427419.8 181100.8) NA
#> 16 POINT (427056.3 180949.5) NA
#> 17 POINT (427425.9 181306.9) NA
#> 18 POINT (427616.8 180887.9) NA
#> 19 POINT (427421.5 181057.2) NA
#> 20 POINT (427437.1 181101.5) NA
#> 21 POINT (427067.6 180731.8) NA
#> 22 POINT (426970.8 180950.9) NA
#> 23 POINT (427248.6 181008.8) NA
#> 24 POINT (427362.4 181054.9) NA
#> 25 POINT (426994.6 180980.5) NA
#> 26 POINT (427179.6 181056.1) NA
#> 27 POINT (427391.4 180976.1) NA
#> 28 POINT (427405.9 181266.7) NA
#> 29 POINT (427457.2 181240) NA
#> 30 POINT (427251.2 181295.3) NA
#> 31 POINT (427252 181388.9) NA
#> 32 POINT (427426.1 181222) NA
#> 33 POINT (427375.7 181136.8) NA
#> 34 POINT (427411 180892.5) NA
#> 35 POINT (427160.6 181451.5) NA
#> 36 POINT (427047.2 181195.5) NA
#> 37 POINT (427128.4 181418.1) NA
#> 38 POINT (427553.2 181340) NA
#> 39 POINT (427550.7 180890.4) NA
#> 40 POINT (427331.8 180972.6) NA
#> 41 POINT (427068.6 181185.9) NA
#> 42 POINT (427550.7 180890.4) NA
#> 43 POINT (427182.9 180991.1) NA
#> 44 POINT (427544.8 180915.6) NA
#> 45 POINT (427337.4 180933.7) NA
#> 46 POINT (427532.3 181355) NA
#> 47 POINT (427094.2 181115.1) NA
#> 48 POINT (427434.3 181057.3) NA
#> 49 POINT (427380.3 181183) NA
#> 50 POINT (427235.8 180713) NA
#> 51 POINT (427486.6 180703.4) NA
#> 52 POINT (427530.3 180943.6) NA
#> 53 POINT (427486.6 180703.4) NA
#> 54 POINT (427084.4 181472.1) NA
#> 55 POINT (427152.9 180709.2) NA
#> 56 POINT (427404.1 180720.3) NA
#> 57 POINT (427574.4 180887.4) NA
#> 58 POINT (427431.9 181185.4) NA
#> 59 POINT (427431.1 181221.7) NA
#> 60 POINT (427514.3 180859) NA
#> 61 POINT (427419.8 181100.8) NA
#> 62 POINT (427558.7 181357.8) NA
#> 63 POINT (427453.5 181323.1) NA
#> 64 POINT (427227.7 181372.6) NA
#> 65 POINT (427227.7 181372.6) NA
#> 66 POINT (427486.6 180703.4) NA
#> 67 POINT (427012.1 180776.6) NA
#> 68 POINT (427489.8 180918.3) NA
#> 69 POINT (427538.5 180980) NA
#> 70 POINT (426998.5 180818.3) NA
#> 71 POINT (427498.1 181171.3) NA
#> 72 POINT (427486.6 180703.4) NA
#> 73 POINT (427498.1 181171.3) NA
#> 74 POINT (427537.9 181350.9) NA
#> 75 POINT (427419.9 181100) 9952
#> 76 POINT (427419.9 181100) 35902
#> 77 POINT (426955 181050.2) 107675
#> 78 POINT (426969.6 180842.9) 137896
#> 79 POINT (427055.6 180795.5) 146984
#> 80 POINT (427055.6 180795.5) 164251
#> 81 POINT (427213.4 181046.9) 181572
#> 82 POINT (427431.3 181220.8) 202607
#> 83 POINT (427426.1 181306.1) 202612
#> 84 POINT (427617.1 180885.2) 249419
#> 85 POINT (427259 181209.2) 273594
#> 86 POINT (427204.7 181173.5) 342477
#> 87 POINT (427114.2 180707.1) 371806
#> 88 POINT (427454.6 181305.5) 374388
#> 89 POINT (427454.6 181305.5) 374485
#> 90 POINT (427373.7 181099) 377533
#> 91 POINT (427051.6 180774.8) 383053
#> 92 POINT (427319.1 180932.5) 464217
#> 93 POINT (427051.6 180774.8) 493103
#> 94 POINT (427215.9 181448.5) 494091
#> 95 POINT (427017.8 181200.3) 511162
#> 96 POINT (427375.1 181054.4) 576920
#> 97 POINT (427574.1 181316.1) 597230
#> 98 POINT (427581 181221.2) 604355
#> 99 POINT (427078.5 180681.6) 604893
#> 100 POINT (427248 180873.6) 618416
#> 101 POINT (427346.5 181302.6) 634455
#> 102 POINT (427437.3 181100.6) 656551
#> 103 POINT (427537.2 180797.2) 663950
#> 104 POINT (427471.6 180901.2) 718545
#> 105 POINT (427616.3 181252.9) 719722
#> 106 POINT (427437.3 181100.6) 728477
#> 107 POINT (427620.5 180691.4) 744847
#> 108 POINT (427437.3 181100.6) 758450
#> 109 POINT (427437.3 181100.6) 761652
#> 110 POINT (427488 180888.9) 767254
#> 111 POINT (427359.8 181097.9) 772820
#> 112 POINT (427068.9 180780.6) 784068
#> 113 POINT (427441.6 181019.1) 798397
#> 114 POINT (427437.3 181100.6) 809360
#> 115 POINT (427215.4 181409.8) 816919
#> 116 POINT (427210.5 181411.1) 816923
#> 117 POINT (427068.5 180710.7) 817134
#> 118 POINT (427359.8 181097.9) 826458