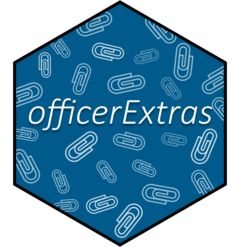
Add a xml string, text paragraph, or gt object at a specified position in a rdocx object
Source:R/add_to_body.R
add_to_body.Rd
Wrappers for officer::body_add_par()
, officer::body_add_gg()
, and
officer::body_add_xml()
that use the cursor_docx()
helper function to
allow users to pass the value and keyword, id, or index value used to place a
"cursor" within the document using a single function. If pos = NULL
,
add_to_body()
calls officer::body_add()
instead of
officer::body_add_par()
. If value is a gt_tbl
object, value is passed as
the gt_object parameter for add_gt_to_body()
.
add_text_to_body()
passes value toglue::glue()
to add support for glue string interpolation.add_gt_to_body()
converts gt tables to OOXML withgt::as_word()
.add_gg_to_body()
adds a caption following the plots using the labels from the plot object.
Usage
add_to_body(
docx,
keyword = NULL,
id = NULL,
index = NULL,
value = NULL,
str = NULL,
style = NULL,
pos = "after",
...,
gt_object = NULL,
call = caller_env()
)
add_text_to_body(
docx,
value,
style = NULL,
pos = "after",
.na = "NA",
.null = NULL,
.envir = parent.frame(),
...
)
add_xml_to_body(docx, str, pos = "after", ..., call = caller_env())
add_gt_to_body(
docx,
gt_object,
align = "center",
caption_location = c("top", "bottom", "embed"),
caption_align = "left",
split = FALSE,
keep_with_next = TRUE,
pos = "after",
tablecontainer = TRUE,
...,
call = caller_env()
)
add_gg_to_body(
docx,
value,
caption = "title",
caption_style = style,
autonum = NULL,
style = "Normal",
pos = "after",
...
)
add_value_with_keys(docx, value, ..., .f = add_text_to_body)
add_str_with_keys(docx, str, ..., .f = add_xml_to_body)
Arguments
- docx
A rdocx object.
- keyword, id
A keyword string used to place cursor with
officer::cursor_reach()
or bookmark id withofficer::cursor_bookmark()
. Defaults toNULL
. If keyword or id are not provided, the gt object is inserted at the front of the document.- index
A integer matching a doc_index value appearing in a summary of the docx object created with
officer::docx_summary()
. If index is for a paragraph value, the text of the pargraph is used as a keyword.- value
object to add in the document. Supported objects are vectors, data.frame, graphics, block of formatted paragraphs, unordered list of formatted paragraphs, pretty tables with package flextable, 'Microsoft' charts with package mschart.
- str
a wml string
- style
paragraph style name. These names are available with function styles_info and are the names of the Word styles defined in the base document (see argument
path
from read_docx).- pos
where to add the new element relative to the cursor, one of "after", "before", "on".
- ...
Additional parameters passed to
officer::body_add_par()
,officer::body_add_gg()
, orofficer::body_add()
.- gt_object
A gt object converted to an OOXML string with
gt::as_word()
then passed toadd_xml_to_body()
as str parameter. Required foradd_gt_to_body()
.- call
The execution environment of a currently running function, e.g.
caller_env()
. The function will be mentioned in error messages as the source of the error. See thecall
argument ofabort()
for more information.- .na
[
character(1)
: ‘NA’]
Value to replaceNA
values with. IfNULL
missing values are propagated, that is anNA
result will causeNA
output. Otherwise the value is replaced by the value of.na
.- .null
[
character(1)
: ‘character()’]
Value to replace NULL values with. Ifcharacter()
whole output ischaracter()
. IfNULL
all NULL values are dropped (as inpaste0()
). Otherwise the value is replaced by the value of.null
.- .envir
[
environment
:parent.frame()
]
Environment to evaluate each expression in. Expressions are evaluated from left to right. If.x
is an environment, the expressions are evaluated in that environment and.envir
is ignored. IfNULL
is passed, it is equivalent toemptyenv()
.- align
Table alignment
scalar<character>
// default:"center"
An option for table alignment. Can either be
"center"
,"left"
, or"right"
.- caption_location
Caption location
singl-kw:[top|bottom|embed]
// default:"top"
Determines where the caption should be positioned. This can either be
"top"
,"bottom"
, or"embed"
.- caption_align
Caption alignment
Determines the alignment of the caption. This is either
"left"
(the default),"center"
, or"right"
. This option is only used whencaption_location
is not set as"embed"
.- split
Allow splitting of a table row across pages
scalar<logical>
// default:FALSE
A logical value that indicates whether to activate the Word option
Allow row to break across pages
.- keep_with_next
Keeping rows together
scalar<logical>
// default:TRUE
A logical value that indicates whether a table should use Word option
Keep rows together
.- tablecontainer
If
TRUE
(default), add tables inside of a tablecontainer tag that automatically adds a table number and converts the gt title into a table caption. This feature is based on code from the gto package by Ellis Hughes to transform the gt_object to OOXML and insert the XML into the docx object.- caption
Name of the ggplot2 label to use as a caption if plot passed to value has a label for this value. Defaults to "title".
- caption_style
Passed to style for
officer::body_add_caption()
. Defaults to same value as style.- autonum
an object generated with function run_autonum
- .f
Any function that takes a docx and value parameter and returns a rdocx object. A keyword parameter must also be supported if named is TRUE. Defaults to
add_text_to_body()
.
Details
Using add_value_with_keys()
or add_str_with_keys()
add_value_with_keys()
supports value vectors of length 1 or longer. If
value is named, the names are assumed to be keywords indicating the cursor
position for adding each value in the vector. If value is not named, a
keyword parameter with the same length as value must be provided. When named = FALSE
, no keyword parameter is required. Add add_str_with_keys()
works
identically but uses a str parameter and .f defaults to add_xml_to_body()
.
Note, as of July 2023, both add_value_with_keys()
and add_str_with_keys()
are superseded by vec_add_to_body()
.
Author
Ellis Hughes ellis.h.hughes@gsk.com (ORCID)
Examples
if (rlang::is_installed("gt")) {
docx_example <- read_docx_ext(
filename = "example.docx",
path = system.file("doc_examples", package = "officer")
)
tab_1 <-
gt::gt(
gt::exibble,
rowname_col = "row",
groupname_col = "group"
)
add_gt_to_body(
docx_example,
tab_1,
keyword = "Title 1"
)
tab_str <- gt::as_word(tab_1)
add_str_with_keys(
docx_example,
str = c("Title 1" = tab_str, "Title 2" = tab_str)
)
}
#> rdocx document with 22 element(s)
#>
#> * styles:
#> Normal heading 1 heading 2
#> "paragraph" "paragraph" "paragraph"
#> Default Paragraph Font Normal Table No List
#> "character" "table" "numbering"
#> Heading 1 Char Heading 2 Char Light Shading
#> "character" "character" "table"
#> List Paragraph header Header Char
#> "paragraph" "paragraph" "character"
#> footer Footer Char
#> "paragraph" "character"
#>
#> * Content at cursor location:
#> row_id is_header cell_id text col_span row_span content_type
#> 1.1 1 TRUE 1 1 1 table cell
#> 1.9 2 FALSE 1 grp_a 8 1 table cell
#> 1.17 3 FALSE 1 row_1 1 1 table cell
#> 1.25 4 FALSE 1 row_2 1 1 table cell
#> 1.33 5 FALSE 1 row_3 1 1 table cell
#> 1.41 6 FALSE 1 row_4 1 1 table cell
#> 1.49 7 FALSE 1 grp_b 8 1 table cell
#> 1.57 8 FALSE 1 row_5 1 1 table cell
#> 1.65 9 FALSE 1 row_6 1 1 table cell
#> 1.73 10 FALSE 1 row_7 1 1 table cell
#> 1.81 11 FALSE 1 row_8 1 1 table cell
#> 2.2 1 TRUE 2 num 1 1 table cell
#> 2.10 2 FALSE 2 <NA> 0 1 table cell
#> 2.18 3 FALSE 2 1.111e-01 1 1 table cell
#> 2.26 4 FALSE 2 2.222e+00 1 1 table cell
#> 2.34 5 FALSE 2 3.333e+01 1 1 table cell
#> 2.42 6 FALSE 2 4.444e+02 1 1 table cell
#> 2.50 7 FALSE 2 <NA> 0 1 table cell
#> 2.58 8 FALSE 2 5.550e+03 1 1 table cell
#> 2.66 9 FALSE 2 NA 1 1 table cell
#> 2.74 10 FALSE 2 7.770e+05 1 1 table cell
#> 2.82 11 FALSE 2 8.880e+06 1 1 table cell
#> 3.3 1 TRUE 3 char 1 1 table cell
#> 3.11 2 FALSE 3 <NA> 0 1 table cell
#> 3.19 3 FALSE 3 apricot 1 1 table cell
#> 3.27 4 FALSE 3 banana 1 1 table cell
#> 3.35 5 FALSE 3 coconut 1 1 table cell
#> 3.43 6 FALSE 3 durian 1 1 table cell
#> 3.51 7 FALSE 3 <NA> 0 1 table cell
#> 3.59 8 FALSE 3 NA 1 1 table cell
#> 3.67 9 FALSE 3 fig 1 1 table cell
#> 3.75 10 FALSE 3 grapefruit 1 1 table cell
#> 3.83 11 FALSE 3 honeydew 1 1 table cell
#> 4.4 1 TRUE 4 fctr 1 1 table cell
#> 4.12 2 FALSE 4 <NA> 0 1 table cell
#> 4.20 3 FALSE 4 one 1 1 table cell
#> 4.28 4 FALSE 4 two 1 1 table cell
#> 4.36 5 FALSE 4 three 1 1 table cell
#> 4.44 6 FALSE 4 four 1 1 table cell
#> 4.52 7 FALSE 4 <NA> 0 1 table cell
#> 4.60 8 FALSE 4 five 1 1 table cell
#> 4.68 9 FALSE 4 six 1 1 table cell
#> 4.76 10 FALSE 4 seven 1 1 table cell
#> 4.84 11 FALSE 4 eight 1 1 table cell
#> 5.5 1 TRUE 5 date 1 1 table cell
#> 5.13 2 FALSE 5 <NA> 0 1 table cell
#> 5.21 3 FALSE 5 2015-01-15 1 1 table cell
#> 5.29 4 FALSE 5 2015-02-15 1 1 table cell
#> 5.37 5 FALSE 5 2015-03-15 1 1 table cell
#> 5.45 6 FALSE 5 2015-04-15 1 1 table cell
#> 5.53 7 FALSE 5 <NA> 0 1 table cell
#> 5.61 8 FALSE 5 2015-05-15 1 1 table cell
#> 5.69 9 FALSE 5 2015-06-15 1 1 table cell
#> 5.77 10 FALSE 5 NA 1 1 table cell
#> 5.85 11 FALSE 5 2015-08-15 1 1 table cell
#> 6.6 1 TRUE 6 time 1 1 table cell
#> 6.14 2 FALSE 6 <NA> 0 1 table cell
#> 6.22 3 FALSE 6 13:35 1 1 table cell
#> 6.30 4 FALSE 6 14:40 1 1 table cell
#> 6.38 5 FALSE 6 15:45 1 1 table cell
#> 6.46 6 FALSE 6 16:50 1 1 table cell
#> 6.54 7 FALSE 6 <NA> 0 1 table cell
#> 6.62 8 FALSE 6 17:55 1 1 table cell
#> 6.70 9 FALSE 6 NA 1 1 table cell
#> 6.78 10 FALSE 6 19:10 1 1 table cell
#> 6.86 11 FALSE 6 20:20 1 1 table cell
#> 7.7 1 TRUE 7 datetime 1 1 table cell
#> 7.15 2 FALSE 7 <NA> 0 1 table cell
#> 7.23 3 FALSE 7 2018-01-01 02:22 1 1 table cell
#> 7.31 4 FALSE 7 2018-02-02 14:33 1 1 table cell
#> 7.39 5 FALSE 7 2018-03-03 03:44 1 1 table cell
#> 7.47 6 FALSE 7 2018-04-04 15:55 1 1 table cell
#> 7.55 7 FALSE 7 <NA> 0 1 table cell
#> 7.63 8 FALSE 7 2018-05-05 04:00 1 1 table cell
#> 7.71 9 FALSE 7 2018-06-06 16:11 1 1 table cell
#> 7.79 10 FALSE 7 2018-07-07 05:22 1 1 table cell
#> 7.87 11 FALSE 7 NA 1 1 table cell
#> 8.8 1 TRUE 8 currency 1 1 table cell
#> 8.16 2 FALSE 8 <NA> 0 1 table cell
#> 8.24 3 FALSE 8 49.950 1 1 table cell
#> 8.32 4 FALSE 8 17.950 1 1 table cell
#> 8.40 5 FALSE 8 1.390 1 1 table cell
#> 8.48 6 FALSE 8 65100.000 1 1 table cell
#> 8.56 7 FALSE 8 <NA> 0 1 table cell
#> 8.64 8 FALSE 8 1325.810 1 1 table cell
#> 8.72 9 FALSE 8 13.255 1 1 table cell
#> 8.80 10 FALSE 8 NA 1 1 table cell
#> 8.88 11 FALSE 8 0.440 1 1 table cell