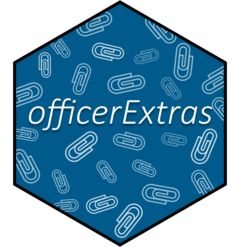
Add a vector of objects to a rdocx object using add_to_body()
Source: R/vec_add_to_body.R
vec_add_to_body.Rd
vec_add_to_body()
is a vectorized variant add_to_body()
that allows users
to supply a vector of values or str inputs to add multiple blocks of text,
images, plots, or tables to a document. Alternatively, the function also
supports adding a single object at multiple locations or using multiple
different styles. All parameters are recycled using
vctrs::vec_recycle_common()
so inputs must be length 1 or match the length
of the longest input vector. Optionally, the function can apply a separator
between each element by passing a value to add_to_body()
or passing docx to
a function, such as officer::body_add_break()
.
Arguments
- docx
A rdocx object.
- ...
Arguments passed on to
add_to_body
gt_object
A gt object converted to an OOXML string with
gt::as_word()
then passed toadd_xml_to_body()
as str parameter. Required foradd_gt_to_body()
.keyword,id
A keyword string used to place cursor with
officer::cursor_reach()
or bookmark id withofficer::cursor_bookmark()
. Defaults toNULL
. If keyword or id are not provided, the gt object is inserted at the front of the document.index
A integer matching a doc_index value appearing in a summary of the docx object created with
officer::docx_summary()
. If index is for a paragraph value, the text of the pargraph is used as a keyword.call
The execution environment of a currently running function, e.g.
caller_env()
. The function will be mentioned in error messages as the source of the error. See thecall
argument ofabort()
for more information.value
object to add in the document. Supported objects are vectors, data.frame, graphics, block of formatted paragraphs, unordered list of formatted paragraphs, pretty tables with package flextable, 'Microsoft' charts with package mschart.
style
paragraph style name. These names are available with function styles_info and are the names of the Word styles defined in the base document (see argument
path
from read_docx).str
a wml string
pos
where to add the new element relative to the cursor, one of "after", "before", "on".
- .sep
A bare function, such as officer::body_add_break or another object passed to
add_to_body()
as the value parameter.- .pos
String passed to pos parameter if
add_to_body()
with .sep if .sep is not a function. Defaults to "after".- .size
Desired output size.
- .call
The execution environment of a currently running function, e.g.
caller_env()
. The function will be mentioned in error messages as the source of the error. See thecall
argument ofabort()
for more information.
Examples
docx_example <- read_officer()
docx_example <- vec_add_to_body(
docx_example,
value = c("Sample text 1", "Sample text 2", "Sample text 3"),
style = c("heading 1", "heading 2", "Normal")
)
docx_example <- vec_add_to_body(
docx_example,
value = rep("Text", 5),
style = "Normal",
.sep = officer::body_add_break
)
officer_summary(docx_example)
#> # A tibble: 13 × 6
#> doc_index content_type style_name text level num_id
#> <int> <chr> <chr> <chr> <dbl> <int>
#> 1 1 paragraph NA "" NA NA
#> 2 2 paragraph heading 1 "Sample text 1" NA NA
#> 3 3 paragraph heading 2 "Sample text 2" NA NA
#> 4 4 paragraph Normal "Sample text 3" NA NA
#> 5 5 paragraph Normal "Text" NA NA
#> 6 6 paragraph NA "" NA NA
#> 7 7 paragraph Normal "Text" NA NA
#> 8 8 paragraph NA "" NA NA
#> 9 9 paragraph Normal "Text" NA NA
#> 10 10 paragraph NA "" NA NA
#> 11 11 paragraph Normal "Text" NA NA
#> 12 12 paragraph NA "" NA NA
#> 13 13 paragraph Normal "Text" NA NA
if (rlang::is_installed("gt")) {
gt_tbl <- gt::gt(gt::gtcars[1:2, 1:2])
# list inputs such as gt tables must be passed within a list to avoid
# issues
docx_example <- vec_add_to_body(
docx_example,
gt_object = list(gt_tbl, gt_tbl),
keyword = c("Sample text 1", "Sample text 2")
)
officer_summary(docx_example)
}
#> # A tibble: 25 × 11
#> doc_index content_type style_name text level num_id row_id is_header cell_id
#> <int> <chr> <chr> <chr> <dbl> <int> <int> <lgl> <dbl>
#> 1 1 paragraph NA "" NA NA NA NA NA
#> 2 2 paragraph heading 1 "Sam… NA NA NA NA NA
#> 3 3 table cell NA "mfr" NA NA 1 TRUE 1
#> 4 3 table cell NA "For… NA NA 2 FALSE 1
#> 5 3 table cell NA "Fer… NA NA 3 FALSE 1
#> 6 3 table cell NA "mod… NA NA 1 TRUE 2
#> 7 3 table cell NA "GT" NA NA 2 FALSE 2
#> 8 3 table cell NA "458… NA NA 3 FALSE 2
#> 9 4 paragraph heading 2 "Sam… NA NA NA NA NA
#> 10 5 table cell NA "mfr" NA NA 1 TRUE 1
#> # ℹ 15 more rows
#> # ℹ 2 more variables: col_span <dbl>, row_span <int>